Let's Workshop: Keyes SHT10
Posted by Sebastian Karam on
Here is a quick introduction to using the Keyes SHT10 Temperature and humidity module. Hopefully it will provide you with the confidence to intergrate climate sensing into your project.
This example will demonstrate the use of an Arduino UNO reading data from the sensor. Once connected and the program loaded, it will read the temperature and humidity, every second. This will use the SHT1x library library created by Jonathan Oxer and Maurice Ribble.
Components
- 1pcs Arduino UNO or Compatible - LCAA100005
- 1pcs Keyes SHT10 Temperature and Humidity Sensor Module - BDAA100025
- 4pcs Male to Female Jumper Cables - GBAA100002
Wiring
Wire the two boards together as can be seen in the image below, taking care to match the pin numbers.
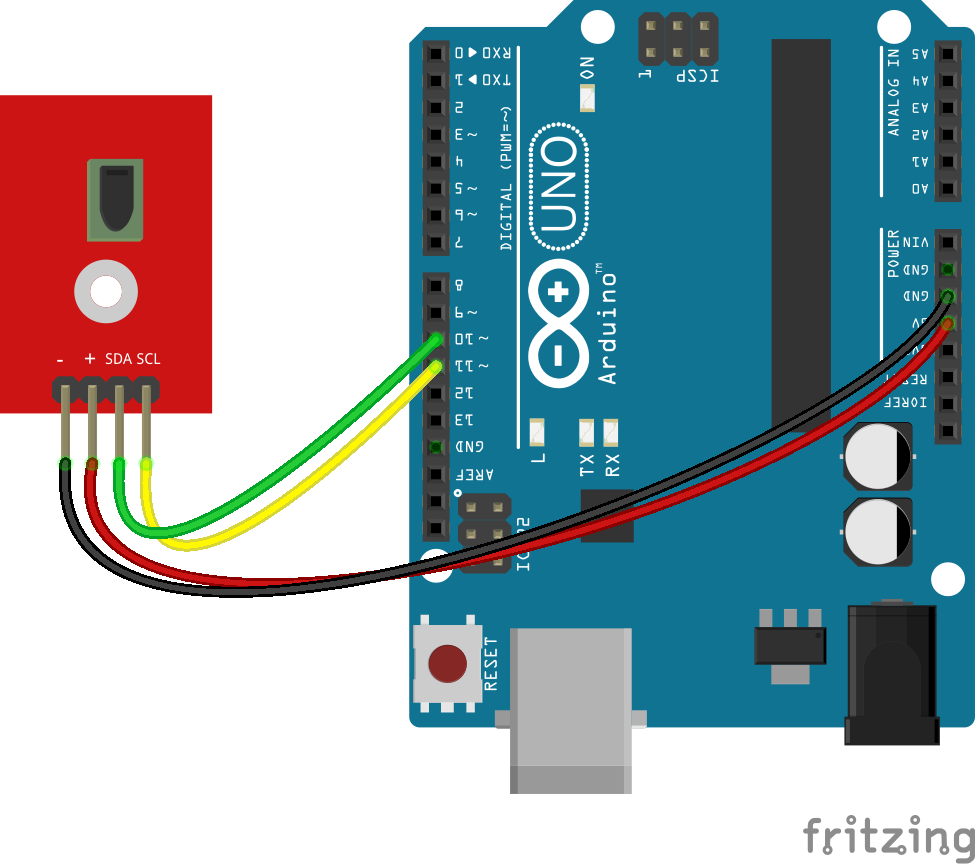
Coding
The code consists of an include, definitions, initilisation, setup and loop. First the SHT1x library is linked to the code. This is followed by the initilisation of the sensor object used by the library and the pins it is connected to. The setup initilises the serial connection. The loop requests the temperature and humidity from the library, storing it in the variables declared. The values are then output to the serial connection, pausing for a moment and repeating.
Load the code below into the Arduino IDE and upload it to your board.
/* A simple program designed to setup and demonstrate the SimpleDHT library and Keyes SHT10 Temperature and Humiditiy Module - BDAA100025 The program uses the SHT1x library to interface with the module and return a temperature and humidity to the serial monitor. Then repeat the request. modified 28 November 2019 by Sebastian Karam - Flux Workshop The SHT1x library created by Jonathan Oxer and Maurice Ribble https://github.com/practicalarduino/SHT1x */ #include// include the SHT1x library // Specify data and clock connections and instantiate SHT1x object #define dataPin 10 // define the data pin the sensor is connected to #define clockPin 11 // define the clock pin the sensor is connected to SHT1x sht1x(dataPin, clockPin); // create the sensor object void setup() { Serial.begin(9600); // initialise the serial connection Serial.println("Flux Workshop Example"); } void loop() { float temperature; // define a variable to store the temperature float humidity; // define a variable to store the humidity // Read values from the sensor temperature = sht1x.readTemperatureC(); // read the values from the SHT10 humidity = sht1x.readHumidity(); // read the values from the SHT10 // Print the values to the serial port Serial.print("Temperature: "); Serial.print(temperature, DEC); // send the result to the serial monitor Serial.print("C / "); Serial.print("Humidity: "); Serial.print(humidity); // send the result to the serial monitor Serial.println("%"); delay(1000); }
Running
With the board loaded with the program and all the connections made, start the serial monitor. An initial value for the temperature and humidity will appear. The output should be as seen below (in this instance, held free, then moist air blown momentarily across it).
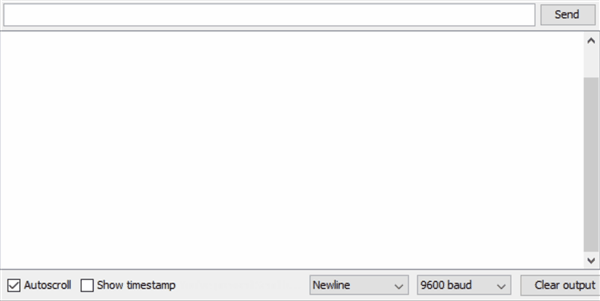
What to try next?
- Output the values to an LCD screen.
- Output the temperature as farenheit instead using exisiting SHT1x library functions.
- Mount the sensor in a sheltered container outside and relay the information on to provide a weather report.
Share this post
- 92 comments
- Tags: Arduino, Humidity, Keyes, SHT10, SHT1x, Steamy Tea, Temperature, UNO
cCPoHExidaA
BDphmHlYZuOf
nayKEfSzZBtQw
QJMHcxGjae
xgCuylkQJBDsZ