Let's Workshop: LC Technology HC-SR04 Ultrasound Module
Posted by Sebastian Karam on
Here is a quick introduction to using the LC Technology HC-SR04 ultrasound module. This will provide you with an entry point to using the module to calculate distance.
The examples will demonstrate the use of an Arduino UNO in controlling the module. The first by producing a pulse then measuring the response directly, then calculating the distance. The second uses the HCSR04 Ultrasonic Distance Sensor library created by Martinsos.
Components
- 1pcs Arduino UNO or Compatible - LCAA100005
- 1pcs LC Technology Ultrasound Module - BDAA100132
- 4pcs Male to Female Jumper Cables - GBAA100002
Wiring
Wire the two boards together as can be seen in the image below, taking care to match the pin numbers.
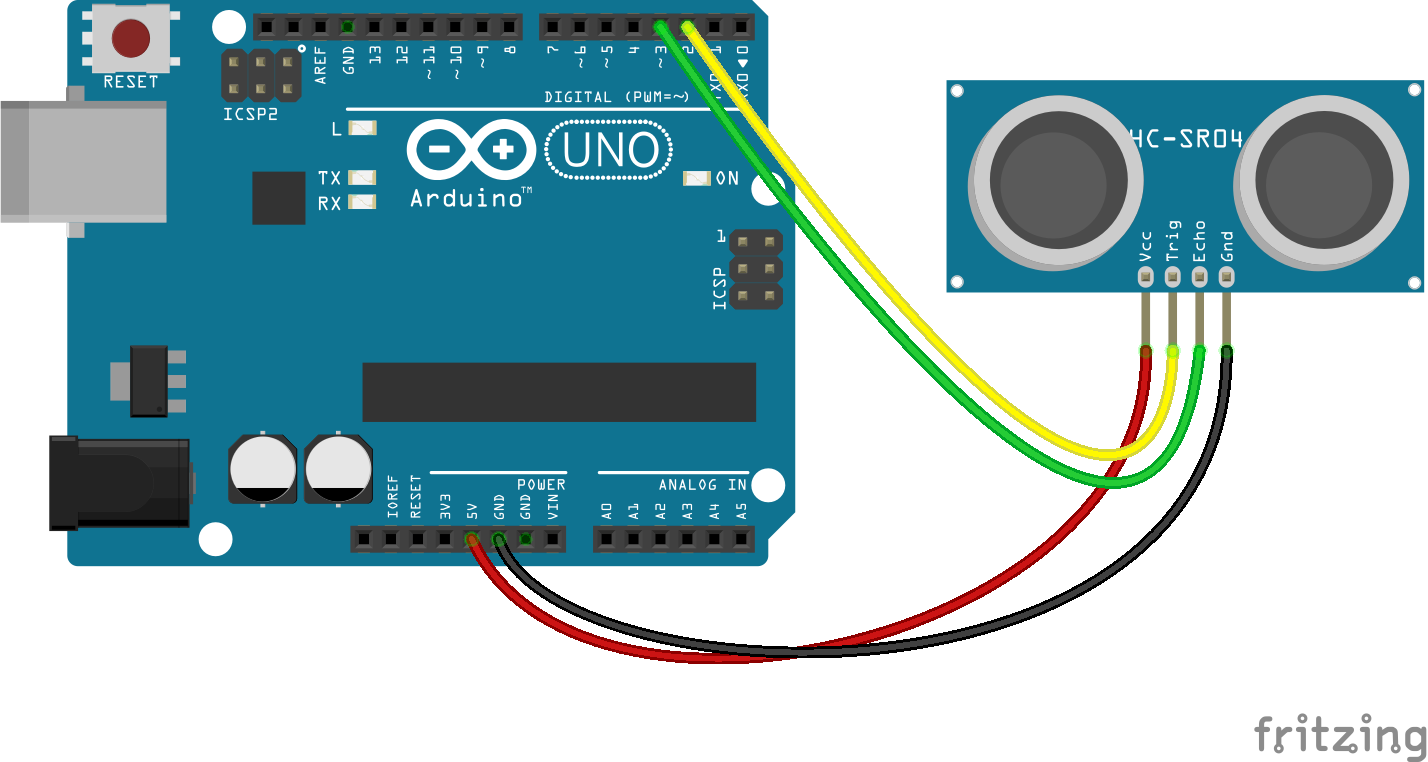
Coding
Example 1
The code consists of definitions, a simple setup and loop. First we define the pins and variables to be used. Next the setup launches the serial monitor, which provides a simple method to debug our output and sets the pin modes. In the loop we first trigger a pulse from the module for 10 microseconds. Then monitor the echo pin for the pulse to return. When a pulse is found, a time is returned. Which is the time taken for the signal to go and then return. With this value we can calculate the distance, as sound can be assumed to travel at a fixed speed. In this case the speed of sound is converted into cm/μs from 340 m/s. Furthermore the time is the time taken for the signal to travel to and back from the target (twice the distance). Following the calculation the result is output to the serial monitor.
Load the code below into the Arduino IDE and upload it to your board.
/* A simple program designed to setup and demonstrate the LC Technology Ultrasound module - BDAA100132 The program pulses the ultrasound module and monitors the return pin for a signal. Then using the time taken for the return to arrive, a distance can be calculated. modified 5th April 2019 by Sebastian Karam - Flux Workshop */ const int trig = 2; // trigger pin number const int echo = 3; // echo pin number long travel_time; // variable to store microseconds float distance; // variable to store calculated distance void setup() { pinMode(trig, OUTPUT); // set the trigger pin as an output pinMode(echo, INPUT); // set the echo pin as an input Serial.begin(9600); // launch the serial monitor Serial.println("Flux Workshop Example 1"); } void loop() { // Sets the trig on HIGH state for 10 micro seconds digitalWrite(trig, HIGH); // set the trigger pin HIGH delayMicroseconds(10); // hold trigger pin high for 10μs digitalWrite(trig, LOW); // set the trigger pin back LOW travel_time = pulseIn(echo, HIGH); // time the duration until the echo pin to go High distance = travel_time * 0.034/2; // calculate the distance given the time taken and speed of sound Serial.println((String)"Distance: " + distance + "cm"); // send the result to the serial monitor delay(1000); // pause for a moment before repeating }
Running
With the board loaded with the program and all the connections made the serial monitor will begin to show the data output by the module. In the example, I move the target from one wall to another and then to face a fuzzy jumper.
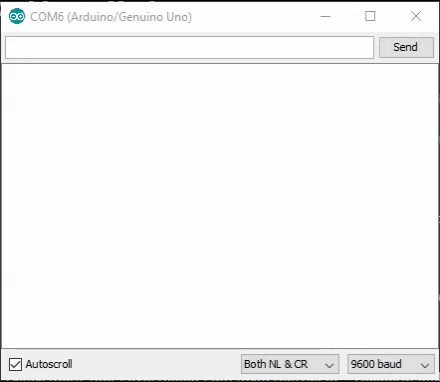
Example 2
The code consists of includes, definitions, a simple setup and loop. First the HCSR04.h library, the HCSR04 Ultrasonic Distance Sensor library. Next we define the pins and variables to be used. Then initialise a sensor class the will interface with the library. Next the setup launches the serial monitor and sets the pin modes. In the loop we simply request the distance from the library in cm. Following the request the result is output to the serial monitor.
Load the code below into the Arduino IDE and upload it to your board.
/* A simple program designed to setup and demonstrate the HCSR04 Library and the LC Technology Ultrasound module - BDAA100132 The program uses the HC-SR04 library to poll the sensor for a distance in cm. modified 5th April 2019 by Sebastian Karam - Flux Workshop HCSR04 Ultrasonic Distance Sensor library created by Martinsos https://github.com/Martinsos/arduino-lib-hc-sr04 */ #includeconst int trig = 2; // trigger pin number const int echo = 3; // echo pin number float distance; // variable to store calculated distance UltraSonicDistanceSensor sensor(trig, echo); // initialise a sensor void setup() { pinMode(trig, OUTPUT); // set the trigger pin as an output pinMode(echo, INPUT); // set the echo pin as an input Serial.begin(9600); // launch the serial monitor Serial.println("Flux Workshop Example 2"); } void loop() { distance = sensor.measureDistanceCm(); // poll the sensor for the distance Serial.println((String)"Distance: " + distance + "cm"); // send the result to the serial monitor delay(1000); // pause for a moment before repeating }
Running
With the board loaded with the program and all the connections made the serial monitor will begin to show the data output by the module. Mimicking the previous example, I move the target from one wall to another and then to face a fuzzy jumper.
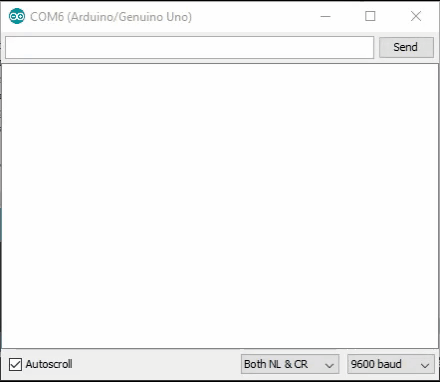
What to try next?
- Use the values to help a robot avoid objects.
- Send the values to an LCD/LED display.
Share this post
- 0 comment
- Tags: Arduino, BDAA100132, Distance, HC-SR04, HCSR04, LC Technology, Ultrasound, UNO